You can keep similar information together by storing an object in the localStorage using JavaScript. We are only allowed to keep strings as key/value pairs in the browser storage like localStorage or sessionStorage.
To store objects in the localStorage, the trick is to convert objects into strings using the JavaScript JSON.stringify()
method. Now save the string with setItem()
method. After getting it from the localStorage, convert it back to an object using JSON.parse()
method.
If you need to save multiple objects together, you can create an array and store the array of objects in localStorage by following the same method.
Let's go through all the necessary steps with some code samples.
List of Methods to Store Objects in localStorage
This is the list of methods required to save objects in the localStorage:
JSON.stringify()
– It converts the JavaScript values like objects or arrays to JSON strings.JSON.parse()
– It converts the JSON strings to JavaScript values based on the strings.setItem()
– It saves a string in the localStorage as a key/value pair.getItem()
– It returns a value from the localStorage related to a key.
How to Store a JavaScript Object in localStorage
I have a user
object with some properties. If you store every property separately in the localStorage, it will be very difficult to manage. That's why I will keep the whole object.
To complete the process you will need 2 JavaScript methods. The JSON.stringify() will convert the object into a string and setItem() will save the converted string in the localStorage.
const user = {
firstName: 'John',
lastName: 'Doe',
email: 'john@example.com',
city: 'New York',
country: 'USA',
};
const userString = JSON.stringify(user);
localStorage.setItem('user', userString);
I am getting the userString
from the conversion process. Then I am saving userString
in the storage under the user
key.
The setItem()
method accepts 2 arguments. The first argument is a unique key and the second argument holds the actual string value.
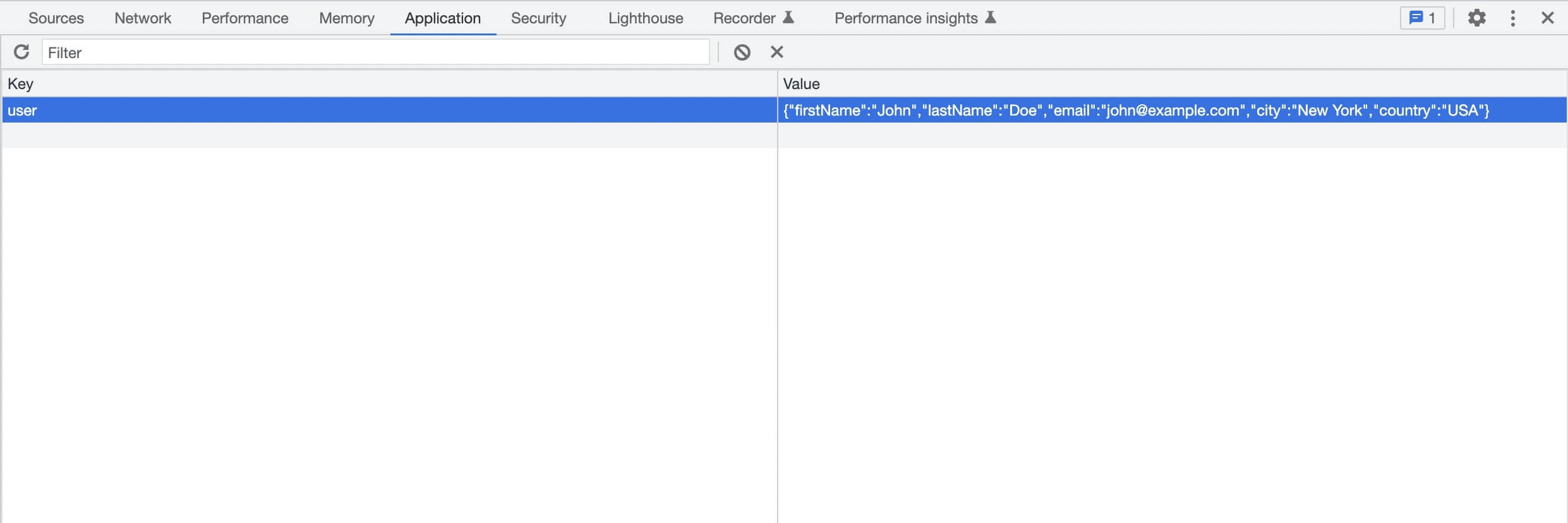
We will use this key to get the value from the localStorage. Because this is how JavaScript will know which value it needs to return.
Also Read: How to Save Images To LocalStorage in JavaScript & Load Them
Getting a JavaScript Object From localStorage
After saving an object in the localStorage, it is easy to get it back. JavaScript has the getItem()
method on the localStorage
object.
This method takes a key as its argument. Then it will return the value associated with that key. You will get null
value if there is no value with that key.
const userString = localStorage.getItem('user');
const user = JSON.parse(userString);
console.log(user);
Output:
{
firstName: 'John',
lastName: 'Doe',
email: 'john@example.com',
city: 'New York',
country: 'USA',
};
What you get from the getItem()
method is a string. To convert it back to the JavaScript object, you have to use the JSON.parse()
method.
You will get the actual user
object by calling the JSON.parse()
method with the userString
string. Now you can use the user
object in your project.
Limitations of This Process
It is not so difficult to save objects in the localStorage and use it in our application later. But this process has some limitations:
- If your object is complex, the
JSON.stringify()
method will not work properly. For example, when the object has methods, it will remove them while converting to the string. - The localStorage has limited storage capacity. It can hold between 2MB to 10MB of data. Therefore, you can't keep as much as you want.
- Users can manually delete data from their browser localStorage. You have to consider it as a temporary storage solution for a limited period of time.
Also Read: Best Ways to Access Object Properties Dynamically in Javascript
Conclusion
In this article, we have seen the process of saving objects in the localStorage. Then we can get it back to use in our application.
There are 2 simple steps to store a JavaScript object in the localStorage. First, convert the object to a JSON string with JSON.stringify()
method. Second, save the converted string in the storage using setItem()
method.