JavaScript objects store data as a key value pair and we can access object properties dynamically by using variables or strings as the keys. You can change those strings or variables programmatically.
The square brackets are used to access object properties dynamically in JavaScript. A property name is defined with a variable or string inside those brackets. This variable or string can be changed based on different conditions. That's why it is known as dynamic.
In this article, we will see different techniques to dynamically access object properties in JavaScript.
How to Dynamically Access Object Properties in JavaScript
Most of the time we use dot notation (obj.property
) to get value from object property in JavaScript. In this way, we get the value by directly using the property name.
The main limitation of this method is its not dynamic. That means you can not change the property name in your code programmatically. Every time you have to type the property name manually.
You can fulfill this need with the square brackets [] instead of using dot notation. You don't have to write the property names every time manually. These names can be changed automatically.
The object property can be accessed dynamically in the following ways:
- Use square bracket syntax in JavaScript.
- Define object property name with a string or variable.
- Access nested object properties using square brackets.
- Use object destructuring to access object properties in JS.
There are many other methods to access object properties in JavaScript when you don't want to do it dynamically.
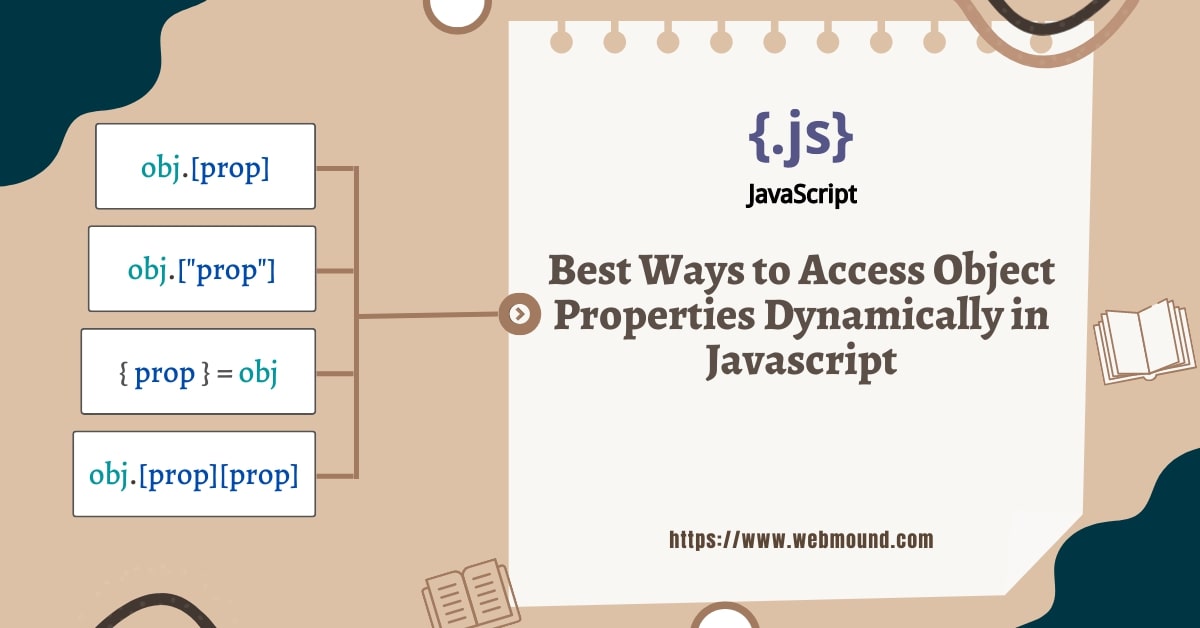
Square Bracket Syntax for JavaScript Objects
This is the most common syntax to access dynamic object properties. The syntax will look like this:
objectName[propertyName]
You have to write the object name followed by square brackets. Inside those brackets, you can define any property name of that object.
For the property name, you can use a string or a variable. You don't have this flexibility in the dot notation.
Also Read: Tricks to Loop Through an Array of Objects in JavaScript
Access Object Properties Dynamically by String in JS
To get value from a JavaScript object dynamically, I will use the square brackets and a string to define the property name. Let's see an example.
const person = {
id: 1,
firstname: 'john',
lastname: 'doe',
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
console.log(person.firstname);
// john
console.log(person['firstname']);
// john
From this person
object, I am accessing the firstname
property. At first, I am using dot notation then I am using square brackets. Both return the same result.
But there are some situations where you can not use dot notation. For example, if the property name contains a dash symbol (-) or space, the dot notation will give an error.
person.first-name
// throws an error
person["first-name"]
// john
You have to use the square brackets in this situation. We will talk about its advantages and when you should access object properties dynamically in the later section in detail.
If you try to access a property that doesn't exist in the object, it will return undefined. You can check whether an object has a property using JavaScript if necessary.
Dynamically Access Object Properties Using Variable
In the square bracket syntax, you are allowed to use variables to get the value out of a JavaScript object. You can change the value of that variable in different conditions and get different properties automatically.
const person = {
id: 1,
firstname: 'john',
lastname: 'doe',
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
const key = 'username';
console.log(person[key]);
// john_doe
You can see I have a key
variable with the value username. I am using the key
variable to access the username
property from the person
object.
If you want to access all the properties from an object, you have to access each property individually like person.id
, person.firstname
etc.
But you can do this automatically by looping through the object in JavaScript and using the square brackets.
const person = {
id: 1,
firstname: 'john',
lastname: 'doe',
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
for (const property in person) {
console.log(person[property]);
}
Output:
1
john
doe
john_doe
john@gmail.com
35
I am looping through the person
object using for...in
loop in JavaScript. Inside the loop, I will have each property name in the property variable.
I can use this variable to get all the property values automatically without accessing them individually.
Dynamically Access Nested Object Properties
You can access nested object properties dynamically in JavaScript using square brackets. You need to use multiple square brackets for a nested object.
const person = {
id: 1,
name: {
firstname: 'john',
lastname: 'doe',
},
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
const key = 'name';
console.log(person[key]['firstname']);
// john
console.log(person['name']['firstname']);
// john
I have a nested object with a name
property that is also an object. The name
property contains two nested properties.
To access these nested properties, I have to use 2 square brackets. One for name
another for nested property names.
You can use string, variable, or both of them together to access a nested object property in JavaScript.
Use Object Destructuring to Access Properties Dynamically
You can use JavaScript object destructuring to access properties dynamically. With this technique, we can extract any object property very easily.
const person = {
id: 1,
firstname: 'john',
lastname: 'doe',
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
const { email, username } = person;
console.log(email);
// john@gmail.com
console.log(username);
// john_doe
I am getting email
and username
properties from the person object with destructuring. You can access as many properties as you want, separated by commas.
It will create the email
and username
variables with the property values. Now you can use those variables to get the values out of them.
Advantages of Accessing Object Properties Dynamically
Even though most of the time, we use dot notation to access properties from an object in JavaScript. But there are some situations where we can't use dot notation.
There are also some advantages of using square brackets. Let's discuss those situations where you have to use square brackets syntax and some of the major advantages with examples.
- The invalid identifier in property name: If the property name has invalid identifiers like dash symbol (-), space, or number at the beginning, you can't use dot notation. That's why you need to use square brackets.
const person = {
id: 1,
'first-name': 'john',
'last name': 'doe',
'2username': 'john_doe',
email: 'john@gmail.com',
age: 35,
};
console.log(person.first-name);
// throws SyntaxError
console.log(person.2username);
// throws SyntaxError
console.log(person['first-name']);
// john
console.log(person['2username']);
// john_doe
As you can see, the person
object contains properties with invalid identifiers. You can not access first-name
, last name
, or 2username
properties with dot notation. It will give a syntax error.
The only way to access those properties is by square bracket syntax. Inside the brackets, you have to define the property name as a string.
- Change property name conditionally: If you are using square bracket syntax, You can change the property name using condition.
const person = {
id: 1,
firstname: 'john',
lastname: 'doe',
username: 'john_doe',
email: 'john@gmail.com',
age: 35,
};
let key = '';
if (condition) {
key = 'lastname';
} else {
key = 'firstname';
}
console.log(person[key]);
If the condition is true, the key
variable will be equal to lastname
. Otherwise, It will be equal to firstname
. We can get different values from person[key]
based on the condition.
- Extract specific properties from an object: By accessing object properties dynamically using destructuring, you can get specific properties from an object.
- Loop through object properties: When we access object properties dynamically using variables, it becomes very easy to loop over the object properties. In this way, we can get all the values without accessing those properties manually.
Also Read: 6+ Ways to Find an Object in an Array of Objects Using JavaScript
When Should Access Object Properties Dynamically
While writing code, most of the time we access property values individually with the dot notation. But you have seen some situations where we can't use it.
We also get some advantages by accessing properties dynamically in some situations. To me, it is good to use both of them combinedly based on the requirements.
Normally, you should use dot notation to get a value from an object. Because it is very easy and widely acceptable in the programming world.
When you need to use variables to get a value from an object, you must get it dynamically. If the property name that you want to access changes for different conditions, you can access that property dynamically.
Conclusion
Getting values from an object using property names is a very common task in JavaScript. The ability to extract values using dynamic object properties gives us more power and flexibility.
We can access object properties dynamically using square brackets syntax. In this way, we can use plain strings or variables to define which property we want to access.
You can access nested object properties and loop through those properties dynamically. While writing code, you often might face this kind of requirement in your application.
That's why it is good to know how to access object properties dynamically in JavaScript along with other common ways.