It is different to loop through an object in JavaScript than looping over an array because JavaScript objects are not iterable. Unlike an array, a JavaScript object contains properties and values.
When we iterate over an object, we can go through its properties, values, or both. We can utilize different methods to handle different purposes.
List of Methods to Loop Through an Object in JavaScript
Before starting the main topic, let me show you the list of methods that we will use throughout this post. Each method will have its separate section where I will show how you can use it with examples.
The methods that can loop through objects in JavaScript are:
- Using the
for...in
statement to loop over an object. - The
Object.keys()
method returns all the properties of an object. - The
Object.values()
method returns all the values of an object. - The
Object.entries()
method returns both the properties and values of an object. - Write a function that loops over JavaScript nested objects recursively.
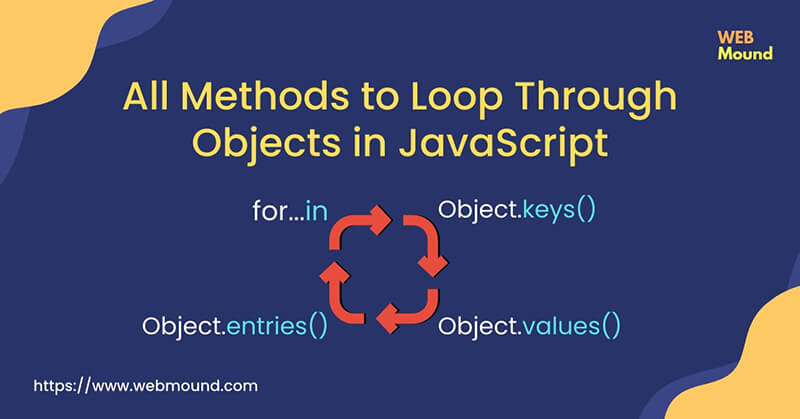
Using for…in To Loop Over an Object in JavaScript
The for...in
statement loops over the properties of an object. For each iteration, it gives one property from the object. By using that key, you can get the value.
This is an example of using for...in
statement to iterate over a javascript object:
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
street: '495 Grove Street',
city: 'New York',
country: 'USA',
};
for (const key in person) {
console.log(`${key} => ${person[key]}`);
}
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
In this example, I am looping over the person
object. Inside the loop, I am getting the keys from this object. This is a very simple solution. But there is one problem that you should keep in mind.
The for...in
statement also loops over the inherited properties in the prototype chain. Objects can inherit properties from their prototypes in JavaScript. Therefore, this statement will iterate over those properties as well.
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
street: '495 Grove Street',
city: 'New York',
country: 'USA',
};
const personExtra = {
gender: 'female',
age: 38,
};
person.__proto__ = personExtra;
for (const key in person) {
console.log(`${key} => ${person[key]}`);
}
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
gender => female
age => 38
In this example, the person
object inherits properties from the personExtra
object. When I use for...in
loop for the person
object, it also prints the inherited properties.
If you want to work with an object's own properties, you have to use the hasOwnProperty()
method. With this method, you can check if a property does belong to an object in JavaScript.
for (const key in person) {
if (person.hasOwnProperty(key)) {
console.log(`${key} => ${person[key]}`);
}
}
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
Inside the for...in loop, I am checking if the property belongs to the person object using the hasOwnProperty()
method. This method accepts the property name and returns true
if that property is present in the object. Overwise, it will return false
.
This time, our loop only prints the properties from the person
object. You can solve the issue by adding a simple if
check inside the for...in
loop.
Also Read: How to Remove Elements From an Array in JavaScript
Using Object.keys() Method to Loop Through an Object
If you only need an object's property names (keys), you can get them by using the Object.keys()
method.
This method takes an object as an argument and returns an array with all the object's properties.
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
street: '495 Grove Street',
city: 'New York',
country: 'USA',
};
const keys = Object.keys(person);
console.log(keys);
// ['first_name', 'last_name', 'phone', 'email', 'street', 'city', 'country'];
You can see that when I pass the person
object to the Object.keys()
method, it returns the keys
array containing all the property names of the person
object.
If you want to get the same output that we had in the previous section, you can use for...of
statement to loop over the keys
array.
for (const key of keys) {
console.log(`${key} => ${person[key]}`);
}
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
You can display properties and values side by side by looping over the array using for...of
statement.
Note: The
Object.keys()
method does not return the names of the inherited properties.
Using Object.values() Method to Loop Through an Object
The Object.values()
method works like the Object.keys()
method. But its output is totally opposite. Where the Object.keys()
method returns the keys, the Object.values()
method in JavaScript returns the values of an object.
This method also takes an object as its argument and returns an array with all the object's values. This method is useful if you only need the list of values of an object.
Note: The
Object.values()
method does not return the values of the inherited properties.
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
street: '495 Grove Street',
city: 'New York',
country: 'USA',
};
const values = Object.values(person);
console.log(values);
Output:
[
'Monica',
'Geller',
'915-996-9739',
'monica37@gmail.com',
'495 Grove Street',
'New York',
'USA',
];
The only problem with this method is you will get the values, but there is no way to access the keys.
But if you want to access both the keys and values at the same this then the next solution is for you.
Also Read: Best Guide on Dynamic Import in JavaScript for Importing Modules
Using Object.entries() Method to Loop Through an Object
The Object.entries()
method accepts an object like the other two methods. But it returns a two-dimensional array. That means each item of the array is also an array.
Each inner array will have 2 elements. The first element is the property name, and the second element is the value.
So, using this method, you are getting the keys and values of a JavaScript object simultaneously.
Note: The
Object.entries()
method doesn't return the keys and values of the inherited properties.
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
street: '495 Grove Street',
city: 'New York',
country: 'USA',
};
const items = Object.entries(person);
console.log(items);
Output:
[
['first_name', 'Monica'],
['last_name', 'Geller'],
['phone', '915-996-9739'],
['email', 'monica37@gmail.com'],
['street', '495 Grove Street'],
['city', 'New York'],
['country', 'USA'],
];
For each property in the object, we are getting an individual array with the property name and the value.
Now we can extract those keys and values using the for...of
loop.
for (const item of items) {
console.log(`${item[0]} => ${item[1]}`);
}
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
By looping over the items array, you can access the keys and values by their index. But if you don't want to access them by index, you can use array destructuring in JavaScript. It will give you the same output.
for (const [key, value] of items) {
console.log(`${key} => ${value}`);
}
With this technique, you give names to the items of an array. When you want to access those items, you can use those names instead of the indexes. It looks very convenient and clean to me.
Also Read: How to Add Hours, Minutes & Seconds to A Date in JavaScript
Loop Through Nested Objects in JavaScript
So far we have seen how to loop over plain objects in JavaScript. That means each property had a single primitive value. But what will happen if some properties contain objects?
So one object will contain another object. How can you loop through a nested object in JavaScript?
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
address: {
street: '495 Grove Street',
city: 'New York',
country: 'USA',
},
};
The person
object has an address property which is an object. If you loop over this person
object just by using for...in
, Object.keys()
, Object.values()
, or Object.entries()
method, it will not return the properties of the address
object.
To get all the properties from the person
object, including the address
object, we must check if any property value is an object inside the loop. You can check using the following function:
const isObject = (val) => {
if (val === null) {
return false;
}
return typeof val === 'object';
};
I have created the isObject()
function, which takes a value as its argument. Then I check if the value is equal to null. Because the type of the null
is an object, you have to return false
explicitly in case of null
value.
Then you can check if the value type is equal to the "object" or not. If it is an object then returns true
otherwise false
.
const nestedObject = (obj) => {
for (const key in obj) {
if (isObject(obj[key])) {
for (const innerKey in obj[key]) {
console.log(`${innerKey} => ${obj[key][innerKey]}`);
}
} else {
console.log(`${key} => ${obj[key]}`);
}
}
};
nestedObject(person);
Output:
first_name => Monica
last_name => Geller
phone => 915-996-9739
email => monica37@gmail.com
street => 495 Grove Street
city => New York
country => USA
I have created nestedObject()
function to loop over an object. This function accepts an object as its argument. Inside the function, I am using for...in
statement to iterate through the object.
In the loop, I have to check if the property value is an object or not using the isObject()
function. If it is an object, it will go through another for...in
loop and display its properties.
But if it is not an object, the else block will run and display the property. This way, we can display all the properties, including the nested objects.
const person = {
first_name: 'Monica',
last_name: 'Geller',
phone: '915-996-9739',
email: 'monica37@gmail.com',
address: {
street: {
street_num: '495',
street_name: 'Grove Street',
},
city: 'New York',
country: 'USA',
},
};
But what will happen if you have an object with double nesting? In a JavaScript program, we might not know the structure of an object.
If your object looks like the person
where we have street
object inside the address
object, the previous solution will not work for this type of object.
Because for each nested object, we have to loop over it. It is practically impossible to add for...in
loop for every nested object because we might not know how many nested objects we have.
const nestedObject = (obj) => {
for (const key in obj) {
if (isObject(obj[key])) {
nestedObject(obj[key]);
} else {
console.log(`${key} => ${obj[key]}`);
}
}
};
nestedObject(person);
To solve this issue, I have to remove the inner for...in
loop and instead I will call the nestedObject()
function with the nested object.
With this change it doesn't matter how many nested objects we have, this function will display all the properties. Whenever our function finds a nested object, it will call itself passing that object.
Also Read: Tricks to Loop Through an Array of Objects in JavaScript
Can You Use forEach(), map(), or for…of Loop On an Object in JavaScript?
You have different methods like forEach()
, map()
, or for..of
to loop through an array in JavaScript. But you can not use these methods on objects.
Because an array is iterable, but an object is not. It is good to use these methods on items that have numeric indexes like arrays. The objects have properties, but they don't have any index value.
That's why we use for...in
, Object.keys()
, Object.values()
, and Object.entries()
methods for only objects in JavaScript. On the other hand, forEach()
, map()
, or for...of
are used for arrays.
Conclusion
You have learned 4 ways to iterate over objects in JavaScript. You can create a function to loop through nested objects. That function automatically will check for nested objects and go through those objects.
The for...in
loop and Object.keys()
method return keys/properties of the object. On the other hand, the Object.value()
method only returns values. You can get both keys and values using the Object.entries()
method.