We loop through an array of objects in JavaScript for many reasons. For example, you can iterate and find an object from an array of objects using JavaScript based on some condition.
The forEach()
method can be used to loop through the array of objects in JavaScript. It gives access to each object present inside an array. There are other statements like for
and for…of
in JavaScript that can do the same thing.
You don't need any special methods or techniques to iterate over an array of objects. You can use the regular JavaScript array methods.
const people = [
{
name: 'john',
age: 35,
},
{
name: 'david',
age: 27,
},
{
name: 'kavin',
age: 35,
},
{
name: 'william',
age: 43,
},
{
name: 'jimmie',
age: 21,
},
];
I will use this "people" array to give you examples though out this article.
You will see 3 ways to loop over this array of objects. You will also learn when you should choose which one at the end of the post.
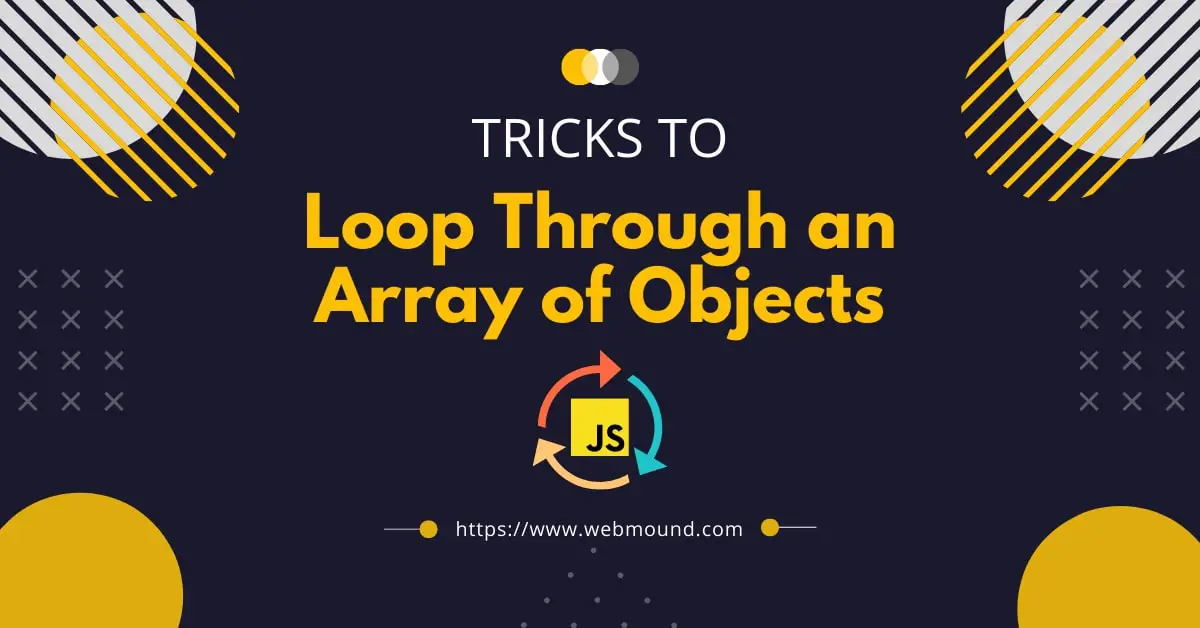
Use forEach() to Loop Over an Array of Objects
The forEach()
method is one of the easiest ways to iterate over an array of objects. This method accepts a callback function and executes that function for each item in the array.
people.forEach((person) => {
for (const key in person) {
console.log(`${key}: ${person[key]}`);
}
});
Output:
name: john
age: 35
name: david
age: 27
name: kavin
age: 35
name: william
age: 43
name: jimmie
age: 21
You also have access to each object and its index as the function parameters. I am looping over the people
array and getting every object in the person
parameter.
Now, you can use this object however you want. I am using the for...in
statement to loop through the object properties in JavaScript.
Use for Loop to Iterate Over an Array of Objects
JavaScript has a traditional the for
statement that can create a loop and give you access to all items inside an array. Therefore, you can use this statement to loop over an array of objects.
for (let i = 0; i < people.length; i++) {
console.log(people[i].name);
}
Output:
john
david
kavin
william
jimmie
It is very easy to the for
statement in JavaScript. You start from index 0 and continue the loop until the index is less than the length of the people
array.
Inside the loop, you have the current index value that can give you the current object. I am printing the name of each person from the array.
But you can perform any operation you want. Like you can shuffle a JavaScript array by using this technique.
Use for…of to Loop Through an Array of Objects
The for...of
statement is used to execute a loop over a JavaScript array. It is similar to the traditional for
statement but has an easy syntax.
It will loop through an array of objects and give you access to the objects directly instead of the index.
for (const person of people) {
console.log(`${person.name} is ${person.age} years old.`);
}
Output:
john is 35 years old.
david is 27 years old.
kavin is 35 years old.
william is 43 years old.
jimmie is 21 years old.
I am creating the loop over the people
array and getting each object in the person
variable. Now, you can check the objects and remove items from the array very easily.
Conclusion
You have seen 3 ways to iterate over an array of objects. The forEach()
method is very easy to use because of its simple syntax. But if you consider the performance then it may not be the best choice.
Note: The traditional
for
statement is much faster than theforEach()
method in JavaScript. If your application needs performace optimization consider usingfor
loop instead of theforEach()
method.
Another advantage of using for
and for...of
is that you can apply the break and continue statements of JavaScript for some conditions.
But as I have seen so far, it really doesn't matter 99% of the time. People use the forEach()
method in most of their applications. So if you are also using this method, it's not the end of the world.
So, you can apply any of these techniques to loop through an array of objects in JavaScript for your application.