Often we add and remove elements from an array in JavaScript. The array is one of the most common data structures in programming. Usually, we use it to store similar types of data.
In real-world applications, we have to add new items, update existing ones and also remove old items from an array. In this article, you will learn how to remove elements from a Javascript array.
So, how can you remove an element or elements from a JavaScript array?
To delete the first element from an array you can use the shift()
JavaScript array method. You can get rid of the last one using the pop()
method and splice()
method for middle items. The JavaScript filter()
method can be used to filter out an array according to the condition that your program may need.
There are many built-in methods and techniques that can be used to eliminate elements from an array in Javascript.
- JavaScript
shift()
method removes an element from the beginning of an array. - JavaScript
pop()
method removes an element from the end of an array. - JavaScript
splice()
method removes elements from the middle or any index of an array. - JavaScript
filter()
method allows removing items conditionally from an array. - Different npm packages like Lodash provide helpful methods that allow updating the JavaScript array.
These are the most common methods and techniques. But You will also learn many other techniques to remove elements from a JavaScript array. Like, deleting elements by id and value, removing objects, removing an item from the middle, removing duplicate values or creating custom functions, etc.
Also Read: In-Depth Guide to Shuffle Any Type of JavaScript Array
How to Remove Elements From The Beginning of a JavaScript Array
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removedElement = langs.shift(); // returns 'HTML'
console.log(removedElement);
// 'HTML'
console.log(langs);
// ['CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
As I have mentioned above, the shift()
is a built-in JavaScript array method that eliminates the first element from an array.
You just have to call this method with an array. This method does not accept any parameter.
Note: This method will change the original array. It does not create any new array.
This method returns the removed element. In the above example, when we use shift()
method with the array langs
, it removes the "HTML" from the original array and returns that element.
It also updates the indexes of other elements in the array and updates the total length of the array. If we call shift()
with an empty array then it returns undefined
How to Remove Elements From The End of a JavaScript Array
Thepop()
method works almost like shift()
method except it removes the last element from an array in JavaScript.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removedItem = langs.pop(); // returns 'PHP'
console.log(removedItem);
// 'PHP'
console.log(langs);
// ['HTML','CSS', 'JavaScript', 'TypeScript', 'NodeJS'];
It also returns the removed element and changes the array length. It modifies the original array.
There is also another way to get rid of the last elements but this is not recommended. You can set the length
value.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
langs2.length = 3;
console.log(langs);
// ['HTML', 'CSS', 'JavaScript']
The array will remove items from the end to match that length. It might be helpful if you need to convert your array into a specific length.
Otherwise, I would recommend you to use the pop()
method to remove elements from the end.
Also Read: Easiest Way to Create Keyboard Shortcuts in JavaScript
How to Remove a Specific Element From an Array in JavaScript
Not always, we remove elements just from the beginning or from the end. Sometimes we have to modify the array by deleting a specific item.
We can achieve this requirement in many different ways. Here I am giving you 4 examples that you will be able to use in almost every situation.
Remove Element Using Array Splice Method by Index
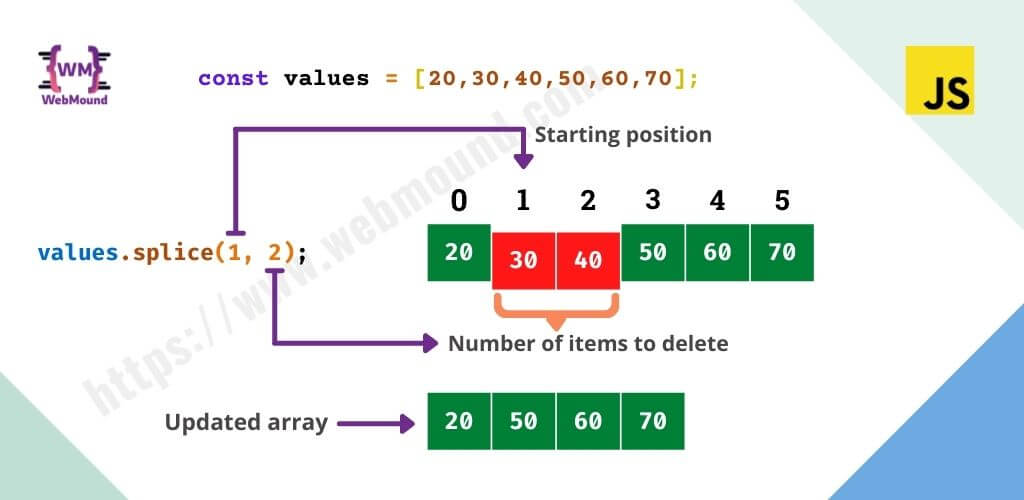
If you know the index of an element that you want to remove then you can use this method. The splice()
is the built-in JavaScript array method.
This method accepts multiple arguments. We use the first 2 arguments to delete items from an array.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removedElements = langs.splice(2, 3);
console.log(removedElements);
// ['JavaScript', 'TypeScript', 'NodeJS']
console.log(langs);
// ['HTML', 'CSS', 'PHP']
The first argument in this method is the index at which to start deleting elements. If we don't provide any arguments then nothing will be deleted.
In the above example, we are passing 2 as the first argument. As JavaScript follows 0 base indexing, here "HTML" is at index 0, "CSS" is at index 1 and "JavaScript" is at index 2.
So, the splice()
method will start deleting from "JavaScript".
In the second argument, we pass the total number of elements we want to delete. We have passed 3 as our second argument. So, 3 elements will be deleted.
Therefore, this method removes "JavaScript", "TypeScript", and "NodeJS" from the array.
It also modifies the original array and returns a new array with the removed items.
Note: The second argument is optonal. If we don't pass anything then it will remove everything from the start index upto the end of the array.
Learn more about - JavaScript splice method
Remove Element Using Array Splice Method by Value
If you don't know the index or want to delete some element by value, you can do that using splice()
method.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
// Finding index
const index = langs.indexOf('NodeJS'); // return 4
const removedElements = langs.splice(index, 1);
console.log(removedElements);
// ['NodeJS']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'PHP']
As we have seen before, the splice()
method requires a starting index. But in this case, we know the value, not the index.
We can find the index from a value using another JavaScript method indexOf()
. When we pass string
as its argument then it returns the index of that string.
If this method does not find the string inside the array then will return -1
.
Learn more about - JavaScript indexOf method
Here I have passed "NodeJS" and it returns index 4.
Now I can use this index in splice(index, 1)
method to remove this element from the array. It will remove 1 element starting from index 4.
Remove Element Using Array Filter Method by Value
Modern JavaScript provides filter()
a helpful array method. Using this method, you can get rid of any element your want. You can use this method instead of splice()
method.
I personally prefer this method over splice() because it provides some features that help to write clean code.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const filtered = langs.filter((element) => {
return element !== 'TypeScript';
});
console.log(filtered);
// ['HTML', 'CSS', 'JavaScript', 'NodeJS', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
This method accepts a callback function as an argument. You can use the traditional function with function
keyword or you can modern arrow function.
This function runs for each element in the array. Every time this function automatically receives three arguments.
The first argument is one element from the array, the second is the index of that element, and the third is the full array.
For this example, we only need the first one.
The filter()
method returns a new array. It does not change the original one. This is one of the important reasons I prefer this method over splice()
.
The call function that we pass in this method only returns true
or false
. If it returns true
then the current element will be included in the new array otherwise it will skip the element.
Learn more about - JavaScript filter method
Here, I want to remove the string "TypeScript". To do so I have to write a condition that returns false
if the current element in the method is "TypeScript".
That's way, I am checking element !== "TypeScript"
. If the current element is not equal to "TypeScript" then the condition will return true
and that element will be included in the new array.
If the current value is equal to "TypeScript" then the condition will return false
and that element will not be included in the new array.
Finally, we get an array that does not contain the string "TypeScript". In this way, we can remove any element from an array.
Remove Element Using Array Filter Method by Index
You can delete any element using the filter()
method by its index. As I have seen, filter()
method callback function may receive 3 arguments.
In the previous example, I used only the first argument. But in this one, I need the second argument that gives the index of the current element.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const filtered = langs.filter((_, index) => {
return index !== 3;
});
console.log(filtered);
// ['HTML', 'CSS', 'JavaScript', 'NodeJS', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
Here, I want to remove the element which is at the index 3
. I am getting the index of the current element as the second argument in the callback function.
Note: Here I have named the first argument as
_
because it is an convention. You can call anything you want. But it is a good practice to name an argument as_
if you have to accept it but you will not use it inside the function.
Then I am checking index !== 3
. If the current index is equal not to 3 then the condition returns true
and that element will be included in the new array.
Otherwise, the condition will return false
and that element will be excluded from the new array. That's how we can remove an element from an array by index.
Remove Object by ID From a JavaScript Array
If the array contains objects, you can loop through an array of objects in JavaScript and remove a specific object from that. You can do this very easily using filter()
method.
const clients = [
{ id: 1, name: 'John', age: 27 },
{ id: 2, name: 'Max', age: 33 },
{ id: 3, name: 'Emma', age: 21 },
];
const filtered = clients.filter((client) => {
return client.id !== 2;
});
console.log(filtered);
// [{id: 1, name: 'John', age: 27}, {id: 3, name: 'Emma', age: 21}]
Here, I have a list of clients. Each client object contains id
, name
, and age
properties. I want to remove a client whose id
is 2.
But I don't know the index of the client object. In a real application, we often face this type of situation. Here, the filter()
is quite useful for such tasks.
Therefore, I am checking client.id !== 2
this condition. This will return false
if the current client id is 2 and the client object will get removed from the new array.
You are not limited to use just id
, you can use any property of an object.
const filtered = clients.filter((client) => {
return client.name !== 'Max';
});
console.log(filtered);
// [{id: 1, name: 'John', age: 27}, {id: 3, name: 'Emma', age: 21}]
You can use the client name
or age
property to write the condition. This will work exactly like the previous one.
Also Read: Factory Function in JavaScript Explained with Examples
Remove Elements From an Array Using Lodash Remove Method
We can use different npm packages to update the JavaScript array. The lodash package provides many helpful methods.
Note: I would not recommend to use this package just to remove elements from an array. Because this package size is quite large. If you need other methods that it provides then you should use it.
There are many methods that you can use just to delete an element from a JavaScript array. For example: filter
, without
, pull
, and remove
.
These methods can be used interchangeably. But they have some differences.
To use this package, first install it in your project. You can follow their official website to know the steps.
const _ = require('lodash');
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const filtered = _.filter(langs, (value) => {
return value !== 'NodeJS';
});
console.log(filtered);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
I have required the package with the name _
. It is a convention but you are free to use any name you want.
The lodash filter()
method accepts 2 arguments. The first one is an array and the second one is a callback function.
This method creates a new array and does not change the original array. When the callback function returns false
, the method skips that item.
const filtered = _.remove(langs, (value) => {
return value !== 'NodeJS';
});
console.log(filtered);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'PHP']
console.log(langs);
// ['NodeJS']
The remove()
looks similar to filter()
in terms of syntax. But the big difference is it updates the original array and the original array only contains the removed elements.
If this method does not find any matching element then it does not remove anything. But it creates a new array with all the elements and the original array becomes empty.
const filtered = _.without(langs, 'NodeJS', 'CSS');
console.log(filtered);
// ['HTML', 'JavaScript', 'TypeScript', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
You can use without()
method to get the same result. In the method, you have to pass the array you want to update and the strings that you want to remove.
You can pass multiple strings separated by comma(,). This method also creates a new array and does not update the original array.
const filtered = _.pull(langs, 'NodeJS', 'CSS');
console.log(filtered);
// ['HTML', 'JavaScript', 'TypeScript', 'PHP']
console.log(langs);
// ['HTML', 'JavaScript', 'TypeScript', 'PHP']
The pull()
method looks similar to without()
in terms of syntax but with one difference. This method modifies the original array.
Remove Element From Middle of an Array in JavaScript
If you need to delete any element from the middle of an array, you can do that. There is no specific JavaScript method for this but I have a solution for you.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
if (langs.length % 2 === 0) {
const index = langs.length / 2;
langs.splice(index, 1);
} else {
const index = Math.floor(langs.length / 2);
langs.splice(index, 1);
}
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'NodeJS', 'PHP']
First, I am checking if the array length is even or odd. If it is even then there will be two items in the middle. You can remove any one of them.
Here, I have an array that has 6 elements. So "JavaScript" and "TypeScript" are in the middle position. I am removing "TypeScript" from these two.
If you want to remove "JavaScript" then just substruct 1 from the index in line number 4
.
const index = langs.length / 2 - 1;
If the array had 5 elements then else
block would run. In this case, there will be one element in the middle. This block of code will remove that middle element.
const langs = ['HTML', 'CSS', 'JavaScript', 'NodeJS', 'PHP'];
const index = Math.floor(langs.length / 2);
langs.splice(index, 1);
Here I have 5 elements in an array. The middle item is "JavaScript" which is at the index 2
.
When I divide the array length by 2, I get 2.5 and Math.floor()
method convert 2.5 to 2. So, I get the index 2.
Now using splice()
method I can remove this item easily. It starts from index 2 and deletes 1 item.
Also Read: Input VS Change VS Blur VS Focus - Differences of JavaScript Events
How to Remove Duplicates From an Array in JavaScript
If you have an array that contains duplicate elements, you can remove those. The following technique will get rid of the elements that are present multiple times.
const langs = ['HTML', 'CSS', 'JavaScript', 'CSS', 'TypeScript', 'NodeJS', 'PHP', 'CSS', 'NodeJS'];
const filtered = langs.filter((value, index) => {
return langs.indexOf(value) === index;
});
console.log(filtered);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
console.log(langs)
// ['HTML', 'CSS', 'JavaScript', 'CSS', 'TypeScript', 'NodeJS', 'PHP', 'CSS', 'NodeJS']
I have an array that contains "CSS" 3 times and "NodeJS" 2 times. This piece of code will keep one "CSS" and one "NodeJS" and remove the others.
How does it work?
First, I have used filter()
method with the original array. This method is getting the current value and index of the current value as its arguments.
Again, I am finding the index of the value
that callback function is getting using indexOf()
method.
Note: The
indexOf()
always returns the index of the first matching element it finds in an array.
For example, the index of the first "CSS" is 1. When it comes to the filter() method, then the callback function receives "CSS" as a value and 1 as its index.
Now inside the callback function indexOf()
method finds the index of the value
that is "CSS" in this case. And this method returns 1 which is equal to the index filter()
method received.
return langs.indexOf(value) === index;
Therefore, this condition returns true
and the current element remains in the new array.
Whenfilter()
gets "CSS" second time, it receives an index which is 3 this time. But when indexOf()
finds the index of the value
it returns 1. Because it returns the first matching elements from an array.
As "CSS" already presents at index 1, this method returns that index. But this time above condition return false
because the calculated index is 1 and the index that filter()
received is 3. They are not equal.
So this element gets removed from the new array. This process repeats for every element in the array.
By using this small piece of code you can remove all the duplicate elements from a JavaScript Array.
Create Custom Function to Remove Elements from a JavaScript Array
Suppose you have to remove elements from the array many times though out your application.
Therefore, you have written the same code over and over again. This is very painful, right? And it is not a good way to write code. We should not repeat the same code.
To solve this issue, you can create your own function. In that function, you will pass the array and the value you want to remove as arguments. The function will do its job and return the result.
You can call the same function as many times as you want in your application. So it solves our problems and makes our life a little easy.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removeElement = (array, item) => {
return array.filter((value) => {
return value.toLowerCase() !== item.toLowerCase();
});
};
console.log(removeElement(langs, 'Javascript'));
// ['HTML', 'CSS', 'TypeScript', 'NodeJS', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
In the above example, I have created a removeElement()
function using filter()
method. This function receives the array and the element I want to delete.
It removes the element and returns the new array. This is an example. You can modify it according to your requirements.
If you like to use splice()
method instead of filter()
, you can do this as well.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removeElement = (array, start, total = 1) => {
return array.splice(start, total);
};
console.log(removeElement(langs, 2, 2));
// ['JavaScript', 'TypeScript']
console.log(langs);
// ['HTML', 'CSS', 'NodeJS', 'PHP']
In the above function, I have used splice()
method. It receives 3 arguments the array, starting index, and the total number of elements I want to delete.
By default, the total number is set to 1, if I don't provide this argument then the function will remove 1 element.
Note: This function returns an array that contains the removed elements and change the original array.
But if you want the behavior like the first function that uses filter()
method then you can use this function.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
const removeElement = (array, start, total = 1) => {
const newArray = [...array];
newArray.splice(start, total);
return newArray;
};
console.log(removeElement(langs, 2, 2));
// ['HTML', 'CSS', 'NodeJS', 'PHP']
console.log(langs);
// ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP']
In the above example, I copy the original array using spread
syntax and create newArray
. When you use splice()
method with the copied array, the original array will not change.
Therefore, this function will return the updated array and the original array will remain the same.
How to Clear or Reset an Array in JavaScript
Sometimes you might need to clear an array. You can remove all the elements from a JavaScript array. I will show you three ways you can achieve this.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
langs.length = 0;
console.log(langs);
// []
You can set the length
property of the array is equal to 0. This will reset your array and make it empty.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
langs.splice(0, langs.length);
console.log(langs);
// []
You can also use the array splice()
method. As starting index, pass 0 in the first argument. This will start deleting from the beginning. In the second argument, I have given langs.length
so it will continue deleting until the array length becomes 0.
Note: If you want, you can remove the second argument. When you provide only the first argument in
splice()
method by default it removes elements upto the end in an array.
const langs = ['HTML', 'CSS', 'JavaScript', 'TypeScript', 'NodeJS', 'PHP'];
while (langs.length) {
langs.pop();
}
console.log(langs);
// []
In the third example, I have used an array pop()
along with while
loop. The while
loop will continue until langs.length
becomes 0.
And each time the pop()
method will remove one element from the end of the array. When all the elements are removed, the array length becomes 0 so while
loop stops.
These are the ways you can clear your array in JavaScript. These are not the only ways. You can achieve this using any other method you want. That's fine.
These 3 methods are also identical in terms of their performance. They take almost the same amount of time to complete.
I have measured their time using console.time()
separately 5 times each. These are the average times, those 3 methods took.
Method 1:
# Reseting the array length
Test 1 - 0.098ms
Test 2 - 0.1ms
Test 3 - 0.099ms
Test 4 - 0.1ms
Test 5 - 0.098ms
-> Average: 0.099ms
Method 2:
# Using splice() method
Test 1 - 0.099ms
Test 2 - 0.098ms
Test 3 - 0.102ms
Test 4 - 0.099ms
Test 5 - 0.106ms
-> Average: 0.1ms
Method 3:
# Using pop() method
Test 1 - 0.105ms
Test 2 - 0.101ms
Test 3 - 0.107ms
Test 4 - 0.105ms
Test 5 - 0.103ms
-> Average: 0.104ms
All the methods took less than a millisecond. But time will vary according to your array size. You can use any one of them it will work absolutely file.
Conclusion
We always use arrays in almost every programming language to store data. Adding and removing items from an array is very important to manage information.
In this article, I have shown you how to remove elements from an array in JavaScript. Now you can create your own custom reusable function using those techniques.