You can modify time by adding hours, minutes, and seconds to a JavaScript Date object. JavaScript has some methods built into this object or you can also use an external library to manipulate the time.
In this post, you will learn everything about all the methods to add hours, minutes, and seconds to Date in JavaScript.
Not only this, but you can also add days to a Date in JavaScript. There are various ways to alter and format a date.
First, let's see the methods we will use in this article.
Methods To Add Hours, Minutes, and Seconds To a Date in JavaScript
I will show you 3 techniques to add time. With each technique, you will be able to add time to your Date object.
- Use vanilla JavaScript to add time to a Date.
- Use the Day.js library to add time to a Date.
- Use the Moment.js library to add time to a Date.
In JavaScript, you have to use setHours()
, setMinutes()
and setSeconds()
methods to modify the Date object. But if you don't want to use 3 separate methods, you have setTime()
to change hours, minutes, and seconds with this one method.
In the case of external libraries, Day.js and Moment.js provide an add()
method. With this one method, you can add any specific amount of time to the Date object. That's why external liberty is very convenient to use in a complex application.
If your application needs a simple date manipulation to display it in a few places, plain JavaScript will be a good choice. But if your application is complex and shows dates and times in multiple places with different formats, you should choose an external library. It will help you to write clean and reusable code.
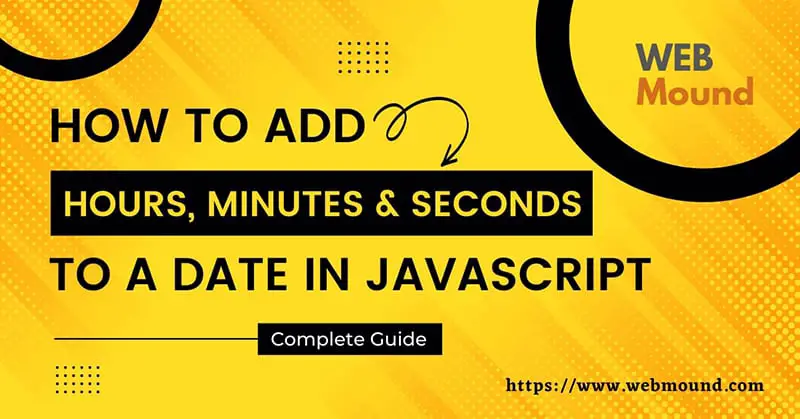
Use Vanilla JavaScript To Add Time To a Date
This section will see how to use pure JavaScript to add hours, minutes, and seconds to the Date object without any external libraries.
How to Add Hours To a JavaScript Date Object
To add hours to a Date in JavaScript, there are 2 methods. You can use setTime()
or setHours()
method.
Using the setTime() method: This method accepts one parameter: time. You have to pass this time in milliseconds. It doesn't matter if you want to add hours or minutes. You have to convert your time into milliseconds before passing it to this method.
const date = new Date();
date.setTime(date.getTime() + 1 * 60 * 60 * 1000);
console.log(date.getTime());
I am creating a Date object using the Date()
constructor function in JavaScript. Now I can call the setTime()
method this object returned from the constructor function. In this example, I am adding 1 hour to the existing time.
But to add 1 hour to the current time, I have to get the current time first from my Date object. I can get the current time using the getTime()
method. This method will return the time in milliseconds.
Also Read: Factory Function in JavaScript Explained with Examples
So, I am getting the current time and then adding 1 hour by converting it into milliseconds to the current time. Finally, I am setting the updated time to the Date object using the setTime()
method.
Using the setHours()
method: If you don't want to go through the hassle of converting hours to milliseconds, you could use the setHours()
method.
This method directly accepts the number of hours that you want to add. There is no need to convert it to milliseconds.
const date = new Date();
date.setHours(date.getHours() + 2);
console.log(date);
After creating a Date object, you can call the getHours()
method on it. This method will return the number of hours currently present in that object.
Add how many hours you want to add to it. In this case, I am adding 2 hours. Finally, pass the updated number to the setHours()
method as the first argument.
Now, if you check your object, you will find that 2 hours have been added to the object.
How to Add Minutes To a JavaScript Date Object
Like the hours, you can also add any amount of minutes to a javascript Date object in 2 methods: with the setTime()
or setMinutes()
method.
Using the setTime()
method: You have to add milliseconds equivalent to the number of minutes you want to add to the current time. Then pass it to the setTime()
method. There is no other difference between adding hours and minutes.
const date = new Date();
date.setTime(date.getTime() + 1 * 60 * 1000);
console.log(date);
I am getting the current time with the getTime()
method after adding 1 minute to it. Finally, I am setting the updated time using the setTime()
method.
As you can see, the process is the same. Just the number of milliseconds you add makes the difference.
Using the setMinutes() method: This method takes the number of minutes that you want to add to the Date object. Pass the number, and it will set that to the object.
const date = new Date();
date.setMinutes(date.getMinutes() + 2);
console.log(date);
I am getting the current number of minutes by calling the getMinutes()
method on the Date object. As I want to add 2 minutes, I am adding 2 with the returned value from that method.
I have to set the total minutes using the setMinutes()
methods.
How to Add Seconds To a JavaScript Date Object
Like the hours and minutes, you can also add seconds by following the same techniques. You can use the setTime()
method or the setSeconds()
method.
Using the setTime()
method: Get the current time using the getTime()
method and add milliseconds equivalent to the total number of seconds you want to add.
const date = new Date();
date.setTime(date.getTime() + 20 * 1000);
console.log(date);
When you get the total milliseconds by adding them with the current time, call setTime()
method to set the updated time to your Date object.
I am adding 20 seconds to my javascript Date object.
Using the setSeconds()
method: Like setHours()
and setMinutes()
methods, you also have setSeconds()
method which sets seconds to the Date object in JavaScript.
const date = new Date();
date.setSeconds(date.getSeconds() + 20);
console.log(date);
I am getting the current number of seconds using the getSeconds()
method. I am adding 20 seconds to it.
Finally, I set the total seconds to the object by calling the setSeconds()
method.
Use Day.js Library To Add Time To a Date
If you don't want to use vanilla javascript to add time to a Date object, you can install the Day.js library in your project. Install this library by running the following command.
npm install dayjs
Now you can use this library to add hours, minutes, and seconds to a Date. You can do all of these just using the add()
method.
This method accepts 2 parameters. The first parameter is the value and the second parameter is the unit. For example, to add 2 hours, you have to pass 2 in the first parameter and "hour" as the unit in the second parameter.
You can learn about this method and a list of units that you can pass in the official documentation.
How to Add Hours To a Date Using Day.js
Import the Day.js library into your file. It will give a function. You will get an object with various methods when you call that function.
const dayjs = require('dayjs');
let date = dayjs();
date = date.add(2, 'hour');
console.log(date.format());
To add 2 hours, I am calling the add()
method on the object. You have to pass "hour" or the shorthand "h" as the unit.
This method will consider the value as hours when you give this unit.
Also Read: Best Guide on Dynamic Import in JavaScript for Importing Modules
How to Add Minutes To a Date Using Day.js
You have to call the same add()
method with "minute" as the unit to add minutes. You can also use "m", which is the shorthand.
const dayjs = require('dayjs');
let date = dayjs();
date = date.add(20, 'minute');
console.log(date.format());
In this example, I am adding 20 minutes to the Date object.
How to Add Seconds To a Date Using Day.js
To add seconds, all you have to change is the unit. For seconds, you have to use "second" or "s" for the unit inside the add() method.
const dayjs = require('dayjs');
let date = dayjs();
date = date.add(20, 'second');
console.log(date.format());
When you pass "second" to the add()
method, it will add the value as seconds. Here, I am adding 20 seconds to the object.
Use Moment.js Library To Add Time To a Date
Another JavaScript library to work with Date and time is MomentJS. To use it in your project, install the library with the following command:
npm install moment
Like the Day.js library, it also provides the add()
method to add any amount of time to a Date object. This method also accepts 2 parameters.
The first one is the amount and the second one is the unit to describe the time. Check out the official documentation to learn more about this method and the units.
Also Read: Self Invoking Functions in JavaScript - Why Should You Use?
How to Add Hours To a Date Using Moment.js
Import the moment()
function from the Moment.js library. Call this function to get the object with all the properties and methods.
const moment = require('moment');
const date = moment();
date.add(2, 'hours');
console.log(date.format());
You have to call the add()
method with the number of hours you want to add and "hours" or "h" as the unit.
For example, I am adding 2 hours to the object.
How to Add Minutes To a Date Using Moment.js
You need to call the add()
method with "minutes" as the unit or "m" for shorthand. This unit will add the value as minutes to the Date object.
const moment = require('moment');
const date = moment();
date.add(20, 'minutes');
console.log(date.format());
I am passing 20 as the value and "minutes" as the second parameter in the add()
method. It will add 20 minutes to the Date object.
How to Add Seconds To a Date Using Moment.js
There is no difference in terms of syntax. You have to change the unit name to add seconds using the add()
method. You have to use "seconds" for the unit or the "s" as the shorthand.
const moment = require('moment');
const date = moment();
date.add(20, 'seconds');
console.log(date.format());
By giving 20 in the first parameter and "seconds" as the unit in the add()
method, I am adding 20 seconds to the Date object.
Which JavaScript Library Should You Use For Date
I always prefer to use Day.js over the Moment.js library. Because the Day.js library is much smaller in size. If the package size is small, your website will download less code. That's why your website will take less time to load.
You will get all the features you need for your application from this library. Due to its smaller size, Day.js is a great alternative for MomentJS.
But if you want to use Moment.js in your project, you can. There is nothing wrong with that. All I am trying to say is that due to the large package size, there is a chance that this library might slow down your website.
Conclusion
My applications need to manipulate the Date and time in JavaScript. You can change the time on your Date with plain JavaScript. Because you will have methods like setTime()
, setHours()
, setMinutes()
and setSeconds()
in the Date object.
With these methods, you can add any specific amount of time to a JavaScript date. But you can also use external libraries if you want.
You have also learned about Day.js and Moment.js libraries to add hours, minutes, and seconds to a Date object in JavaScript. You can choose any one of them to work with Dates.
I hope you have learned everything about adding and changing time to a JavaScript date from this post.
Thank you so much for reading. Happy coding!