JavaScript has a couple of ways to change and redirect an URL to another page on the client side. There are many reasons why we could decide to redirect our URLs.
The location.href
property, location.assign()
and location.replace()
methods are used to redirect an URL to another page in JavaScript. This property and methods accept an URL and load it by replacing the existing page.
In this article, you will learn how you can use the location
object to redirect any web page using JavaScript. You can use these techniques to go to a new domain or just move to another path in your own domain.
Whatever your requirement is you can do that by following our ways. Let's go through each of them one by one.
Change and Redirect a URL to Another Page in JavaScript
Most of the time we redirect our URLs from the server. Because when we use server-side redirects, we can add HTTP 3xx status codes with them.
By watching those status codes, search engines like Google can understand the reasons behind those redirects. That is why it is good for SEO (Search Engine Optimization).
You use software like Nginx or your application server side. If you are using NodeJS to build your application server side, you can easily redirect an URL from Node.js and Express server.
But if you don't have access to a server or you want your redirect to happen based on the user's action like click etc, you need to use JavaScript on the client side.
All these methods will change the current URL in the browser address bar but it will also refresh the page. You can change an URL dynamically without reloading the page in JavaScript.

Redirect Using the location.href Property
The location.href
property is one of the most common ways to redirect an URL using JavaScript. Usually, we can use this property to get the current URL of a web page.
console.log(window.location.href);
// https://www.example.com/products
We can also use this property to set a new URL. By setting a new URL, we tell the browser to load that new URL by replacing the existing one.
You can set your target URL by adding an equal sign (=) after this property followed by the URL as a string.
window.location.href = "https://www.example.com";
The window.location.href
property not only redirects an URL but also keeps the previous URL in the browser history. That means you can click the "Back" button in your browser to get back to the previous page.
Note: If you set an empty string, this property will reload the current page.
You can set both an absolute and a relative URL using the window.location.href
property. If the URL is not formatted correctly, it will throw an error.

Redirect Using the location.assign() Method
The location.assign()
is a method that functions like the previous property. The main difference between them is that it is a method therefore you need to call it.
This method takes one parameter. You have to pass your target URL in this parameter as a string. If you call this method without passing this parameter, it will give an error.
Note: If you pass an empty sting, it will reload the current page.
This is the syntax of how you can call this method:
window.location.assign("https://www.example.com");
I am calling the assign()
on the location
object in JavaScript with an absolute URL. You can also use a relative URL to change the path.
This method also keeps the previous URL in the browser history. When you change your URLs using this method, you will be able to go back to those previous pages.
As you have seen, both location.href
property and location.assign()
method are pretty much the same in terms of functionality.
Both redirects to a new page by keeping the browser history of its parent page. But the location.assign() method is considered to be a little safer.
Because while using this method if the target URL is broken or not secure, it will throw a DOMException error.
Also Read: How to Open URL Using JavaScript in Same or New Tab/Window
Redirect Using the location.replace() Method
In the JavaScript location object, there is a location.replace()
method that you call to perform JavaScript redirects. This method looks like the previous location.assign()
method but its functionality is different.
The location.replace()
method accepts one parameter where we can specify our target URL. We have to pass this parameter as a string.
Note: If you pass an empty sting, this method will reload the current page.
This is the syntax you need to follow to use this method:
window.location.replace("https://www.example.com");
As you see, you can call the replace()
method on the location object in JavaScript like the assign()
method. But there is a big difference between them.
The difference is that the replace()
method doesn't add a new browser session history for the previous URL. Instead, this method replaces the current entry with the previous one.
That means when you redirect from page A to page B using the location.replace() method, you can't go back to page A by clicking the "Back" button in the browser.
But if you redirect from page A to page B using location.href
property or location.assign()
method, you will be able to go to page A by clicking the "Back" button.
How to Redirect to a Relative URL
In the previous examples, we have seen how we can use those methods to redirect to an absolute URL. We normally use the absolute URL to go to a different domain name than ours.
but what if I want to redirect my users to a different path within my website? I can easily do it using a relative URL.
// Using location.href property
window.location.href = "/blog";
// Using location.assign() method
window.location.assign("/blog");
// Using location.replace() method
window.location.replace("/blog");
Here, I am showing you how to use a relative path with all 3 techniques. When you use any one of these techniques in your code, it will take you to the /blog
page on the same website.
For example, if you are on the https://www.example.com/products
page and redirect to the /blog
page, it will take you to the https://www.example.com/blog
page.
Redirect a Page in JavaScript with parameters
We add parameters to an URL using the ?
and &
signs in JavaScript. Many times we need to use parameters with our URLs to display different information based on the parameter values.
For example, if you have a /user
page where you want to show information about a user. You can pass the username with this URL to know whose information this page should display.
Therefore in order to redirect to those pages, you have to attach parameters with the target URL. Let's see how we can do it.
const url = 'https://www.example.com?username=john37';
// Using location.href property
window.location.href = url;
// Using location.assign() method
window.location.assign(url);
// Using location.replace() method
window.location.replace(url);
In this example, I am adding the username parameter with its value to the target URL. Then I can redirect to this page.
Also Read: How to Check For Hash (#) Value in a URL Using JavaScript
How to Redirect in JavaScript After 5 Seconds
You can redirect a user to a new page after waiting for a fixed amount of time. We could use the setTimeout()
function in JavaScript to achieve this.
For this example, I will wait for 5 seconds and then redirect to a new URL. The time is completely customizable. You can wait for any amount of time.
setTimeout(() => {
window.location.replace("https://www.example.com");
}, 5000);
The setTimeout()
function takes time in milliseconds. I am giving 5000 milliseconds because I want to wait for 5 seconds. If you need more or less time, you can change this value.
Inside the callback function, I am using the location.replace()
method for JavaScript redirects. It is better to use location.replace()
instead of using other methods.
Because it will not create a new entry in the session history for the previous page. If a user clicks the "Back" button and goes back to the previous page, it will again redirect after 5 seconds automatically.
That's why you should use the location.replace()
method so that no one can go back to the previous page.
Redirect to a Page in JavaScript on Button Click
Most of the time we use JavaScript redirects on the client side based on user actions. For example, if a user clicks a button, you will perform some functions and then redirect to a new page.
You can do this by listening to the click event. Inside the callback function, you can perform whatever action you want to perform, and at the end, you will redirect.
const btn = document.getElementById("btn");
btn.addEventListener("click", () => {
// Perform other functions here...
// Using location.href property
window.location.href = "https://www.example.com/dashboard";
// Using location.assign() method
window.location.assign("https://www.example.com/dashboard");
// Using location.replace() method
window.location.replace("https://www.example.com/dashboard");
});
I am getting the HTML button using the getElementById()
method. When a user clicks on this button, I can use any of the 3 methods for the redirection.
How to Redirect in JavaScript on Page Load
If you don't want to show the content of a specific page, you will redirect your users immediately after the page loads. For that, you don't have to do anything extra.
In this situation, you should use location.replace()
method in your JavaScript file and link it inside the <head>
tag. In this way, browsers will redirect immediately without loading the <body>
contents.
If you don't want to use a separate JavaScript file for this, you can write the following code inside the <script>
tag and place that in the <head>
element of your page.
<script>
window.location.replace("https://www.example.com/blog");
</script>
I am using location.replace() method because I don't want my users to come to this page again by clicking the "Back" button in the browser.
If they come back to that page, it will again redirect. It will be a bad user experience.
Also Read: Self Invoking Functions in JavaScript - Why Should You Use?
Redirect on Page Load in HTML
When you want to redirect a page on page load, you can do this using HTML. When we use <meta>
tag with http-equiv="refresh"
attribute, it redirects to a specific URL.
This meta tag also doesn't keep the session history for the previous page in the browser. Users can not go back to the previous page by clicking the "Back" button.
We can specify our target URL in the content attribute. This is the following code you have to add inside your document <head>
element.
<meta http-equiv="refresh" content="0; URL=https://www.example.com">
There are 2 attributes in this <meta>
tag. You have to set the http-equiv
attritube to "refresh" and in the content
attribute, you need to set 2 things.
The first part contains the time delay. I am setting 0 for the time delay. Because I want to redirect without waiting.
If you want to wait for a few seconds, you can specify that time here. For example, to wait for 5 seconds, you will set it as 5.
The second part contains the target URL where I want to redirect. When browsers find this meta tag, they will redirect to the specified page automatically.
Which Method Should You Use?
The techniques that we have seen in this article look very similar. But there is a little difference between them. Therefore, before choosing one of them you should keep this difference in your mind.
Between location.href
and location.assign()
method, you can use any one of them. Because both have the same type of characteristics. They can redirect to a new page by creating a new entry to the browser session history.
If you want to go back to the previous page after redirects, you can use any one of them. But if you don't want to add an entry to the session history in the browser, you have to use the location.replace()
method instead.
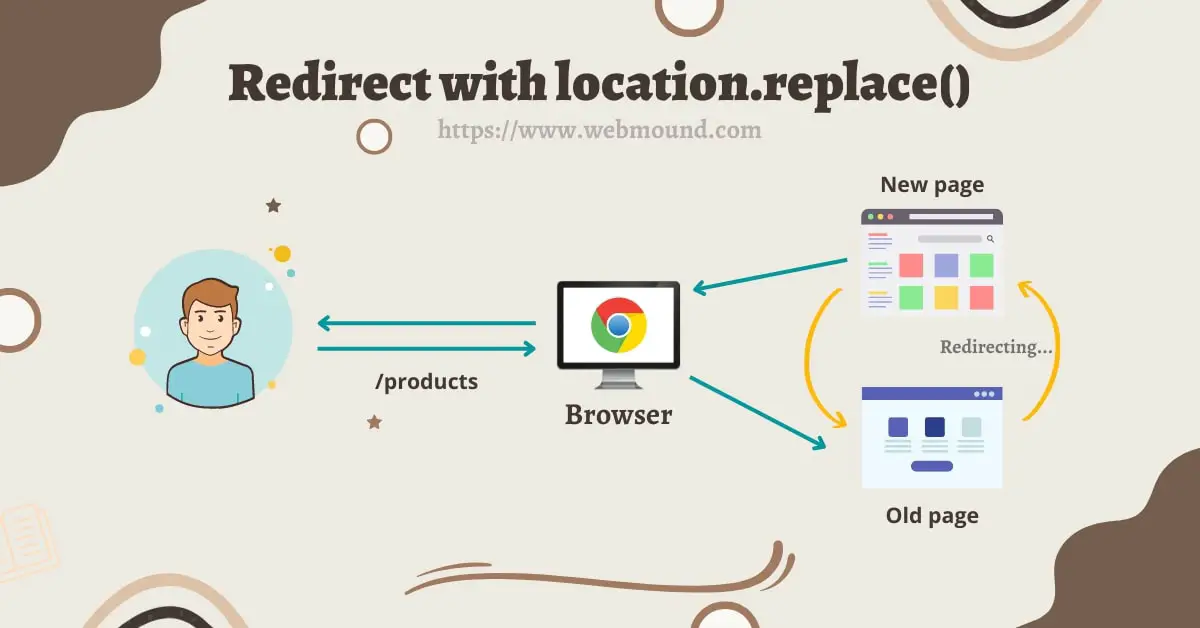
Especially if you redirect to a new URL as soon as the page loads, you should use the location.replace()
method. Otherwise, users will be able to go back to the previous page.
But when they go back to the old page, it will again redirect to the new page. For this reason, users will not be able to use the browser's "Back" button. It is also a very bad user experience.
Conclusion
You have learned about the location.href
property, location.assign()
and location.replace()
methods. Each of them helps us to perform JavaScript redirects.
Even though their functions are the same, they also have some differences. That's why they are a good fit for different situations. I have shown you where you should use which method.
I hope this article was helpful for you and you have understood this topic. Whenever you need to redirect an URL to another page using JavaScript, you can use these techniques.