Self-invoking functions (SIFs) are a powerful JavaScript feature that lets you execute code automatically. In this article, we'll explore why you should use SIFs and how to create them. We'll also provide a few example implementations to see how they work.
What is a Self Invoking (Executing) Function in JavaScript?
A self-invoking function is a function that executes automatically when it is defined. In other words, it calls itself. An anonymous function is defined inside a set of parentheses followed by another set of parentheses. It is also known as an anonymous executing function or an immediately invoked function expression (IIFE).
There are different types of functions available in JavaScript. Like regular function, an anonymous function, factory function, constructor function, etc. But a self-executable function behaves differently than the rest.
Even though people call it by different names, its purpose or syntax is the same. You can write a self-executable anonymous function very easily and it is also simple to use.
To understand this type of function, how it works, and the differences from a normal function, you should know about the function declaration and execution.
function myFunction () {
// write you code
}
This is known as the function declaration. We use the function
keyword and give a name for the function. We write our code in between the curly brackets as the function body. You can also use the arrow function if you want.
But when we write a function like this, it doesn't get executed. Declaring a function means saving it for later use. You can use your declared function by calling it with the function name followed by a set of parentheses.
myFunction();
Now, I am invoking or executing the myFunction()
like this. A normal function must be executed by calling its name.
If a function doesn't have a name, it is called an anonymous function. As a self-invoking function doesn't have to call explicitly with a name, we can use a nameless (anonymous) function.
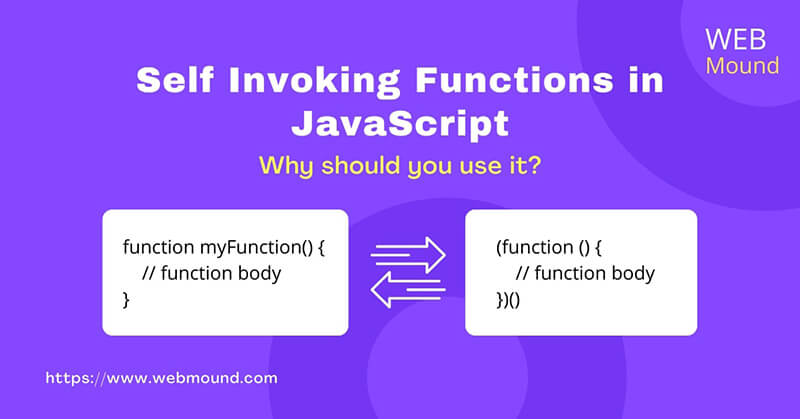
How to Write a Self Invoking Function in JavaScript
There are 2 types of syntax that you can follow to write a self-executing function in JavaScript. There are:
(function () {
// function body
}());
The alternative syntax:
(function () {
// function body
})();
// Arrow function
(() => {
// function body
})();
The second syntax is more popular and widely used than the first one. Another difference between them is you can use the arrow function with the second syntax. But if you try to use arrow function with the first syntax, it will give you an error.

I always prefer the second syntax over another. Most of the time you will find people using this one instead of the first one. Because it is easy to write and also looks very clean and simple.
// --- First step
() ()
// --- Second step
(function () {
// function body
}) ()
First, type two sets of parentheses side by side. Second, write your function in between the first set of parentheses. The second set of parentheses will work as function execution.
That's it. You can write a self-invoking function in JavaScript with these 2 simple steps.
How Self Invoking Functions work in JavaScript
You already know in JavaScript, a self-invoking function can be invoked (executed) without being called. When a JavaScript engine finds a function like this, it immediately runs the code inside the function.
A JavaScript engine executes this function just once after the declaration. As this function is anonymous, it does not keep a reference to that function
After this function gets executed, there will be no reference of this function in the memory like a regular function. Therefore, you can not use this function a second time, not even its return value.
Also Read: Best Ways to Create Dynamic HTML Element & CSS in JavaScript
Example of a Self Executing Function in JavaScript
We have seen syntax and how self-executing functions work. Now let's see an example with a real-world use case.
Suppose you have a web page where you display the list of names. It can be anything. In this example, I am showing a list of programming language names.
const languages = ['Python', 'PHP', 'Go', 'Rust', 'C++', 'Swift', 'Java', 'JavaScript'];
You display these names on your web page. But you want to show these name in a different order each time a user reload the page. That means you need to shuffle the array in JavaScript when your web page loads.
This is a perfect place to use a self-executing anonymous function in JavaScript because you have to shuffle your array just once when your page loads.
const languages = ['Python', 'PHP', 'Go', 'Rust', 'C++', 'Swift', 'Java', 'JavaScript'];
(() => {
languages.reverse().forEach((item, index) => {
const j = Math.floor(Math.random() * (index + 1));
[languages[index], languages[j]] = [languages[j], languages[index]];
});
})();
console.log(languages);
// [ 'Rust', 'Python', 'Swift', 'PHP', 'Java', 'C++', 'Go', 'JavaScript' ]
Here, I have created a self-executable function. When the page loads, this function will automatically be executed and shuffle the array. So, each time we will get the languages array in a different order.
How to Pass Parameters in a Self Invoking Function in JavaScript
In the previous example, you have used the languages
array directly inside the self-executing function. But you can pass the array as a parameter if you want.
A self-executing function can accept parameters when it gets executed. It is very easy to set up.
const languages = ['Python', 'PHP', 'Go', 'Rust', 'C++', 'Swift', 'Java', 'JavaScript'];
((list) => {
list.reverse().forEach((item, index) => {
const j = Math.floor(Math.random() * (index + 1));
[list[index], list[j]] = [list[j], list[index]];
});
})(languages);
console.log(languages);
// [ 'JavaScript', 'Java', 'Swift', 'PHP', 'C++', 'Python', 'Rust', 'Go' ]
This time inside our self-invoking anonymous function, we are not using the languages
array directly. Instead, I am passing this array through the second set of parentheses and receiving the array as a list
in the anonymous function.
You can pass as many parameters as you want to this function by adding them between these parentheses separated by a comma (,). You will receive those parameters in that anonymous function just like a regular function.
Async Self Invoking Functions in JavaScript
You can also use async...await
in self-invoking functions in JavaScript. It is necessary if you want to load some data from the server when a web page loads.
Learn more about async/await
syntax here.
You can define a self-executing function using async
and await
to make an asynchronous server call. When your web page loads, this function automatically will send the request to the server.
(async () => {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await response.json();
console.log(data);
})();
console.log('loading finished');
In this example, I am making an asynchronous call using the fetch()
API in JavaScript. This returns a promise to I am using async
and await
here.
When we execute this function on a webpage, you will see that the second console.log()
will show first because our self-executing function will run asynchronously. After that it will load the data.
Also Read: Synchronous VS Asynchronous Programming with Examples
Difference between Self-Invoking Function and Normal Function
We will immediately notice a few differences if we look at a self-invoking function and a normal function because there are some differences in terms of their syntax or their behavior.
- We can use a function name for a regular function or define it as a nameless (anonymous) function. But we always use an anonymous function as a self-executing function.
- They also look different because of their syntax. A normal function uses the function keyword, or it defines as an arrow function without any extra parentheses. But we always declare a self-invoking function between a set of parentheses followed by another set of parentheses.
- A normal function can never execute itself. There are 2 steps: declaration and execution. First, we declare a function and then execute it by calling it its name. But in the case of a self-invoking function, both steps happen simultaneously. It gets executed immediately after its declaration. You don't have to call it like a regular function.
But there is no difference when a JavaScript engine executes the function body (code inside the function), except for how they are called and their syntax.
Usages of Self Executing Functions in JavaScript
Self-executing functions are a great way to run a piece of code instantly, just once. You can also organize your code and create a separate module using a code snippet.
They're also really useful for testing purposes - you can easily create a small self-executing function to test a single feature or line of code without worrying about global variables or other side effects.
It is a great candidate when you want to declare a function and execute it without explicitly calling it.
Use this type of function if you want to run it one time without keeping the reference. After its execution, the JavaScript engine will remove everything related to this function from memory.
Advantages of Self Invoking Functions in JavaScript
After knowing about self-invoking functions and their syntax, one question might come to your mind: What advantages will you get using a self-invoking function in JavaScript.
To answer this question, I have listed a few advantages for you:
- This function aims to create an artificial namespace that doesn't exist in JavaScript.
- As it is light weighted syntactically compared to a named function, it can be easier to use a self-invoking function if the function is used once.
- Although these functions take arguments, it is not recommended to pass too many arguments. No matter how simple the names of the arguments were, the code would look complicated.
- Because these functions have no identifiers or closures, they are invoked directly without using any identifiers or closures, preserving the function's scope.
- Modular patterns are typically developed using self-invoked functions, in which the reference to the object returned by those functions is kept.
Conclusion
We have learned about the JavaScript self-invoking functions and how we can use them in JavaScript. I have listed a few advantages to provide a brief overview of this feature.
In JavaScript, a self-executing function is just an anonymous function with a different syntax that gets executed one time instantly after it's declaration. It also receives parameters and handles promises using async/await
syntax.
I have demonstrated a few examples of those functions and the differences between Anonymous and Named functions.
You also have seen how you can pass arguments and how to use async-await
with self-executing anonymous functions in JavaScript.
I hope it was helpful. Thank you so much for your time. Happy coding!