The main differences among input
, change
, and blur
events in JavaScript are how and when these events fire.
In JavaScript, we use input, change, and blur events with HTML form elements.
The input
event fires when users enter something in the form elements. On the other hand, the change
event fires when users update the input value and remove focus from the input element by clicking away. Finally, the blur
event fires whenever users focus on the input element and then remove the focus.
In this article, I will show you the Input vs Change vs Blur event in Javascript and all the differences among those events.
Read also: How to Remove Elements From an Array in JavaScript
Input VS Change Event in JavaScript
<input type="text" id='username' placeholder='Enter your username...'>
Here, I have a input
field that has an id
"username". Now I will show you how you can use input and change events using this input field and how these two events are different.
const username = document.getElementById('username');
username.addEventListener('input', () => {
// Write your logic here
console.log('input event');
});
I am selecting the field using getElementById
and adding input
event on it.
Now, whenever I enter something in the input
field, the code inside the function executes.
Every time I press any key on my keyboard this function runs. This also happens if I press the backspace
button.
That means whenever you enter any character or remove any character from an input field this event fires.
const username = document.getElementById('username');
username.addEventListener('change', () => {
// Write your logic here
console.log('change event');
});
But in the case of change
event, this is a little different.
When I enter something in the input
field, this event does not fire. But whenever I click away and remove the focus from the input
field, this event fires, and the function executes.
But if the value in the input
field does not change, this event does not fire even if I focus on the input
field and remove the focus.
Differences Between Input and Change Events in JavaScript
- The
input
event fires for every keypress and thechange
event fires when field value changes and field loses the focus. - We use the
input
event when we need to show updated data to users in real-time. On the other hand, we use the change event when we want to do something when the field value changes. - The
input
event occurs for every change in the value and thechange
event occurs only once when we leave the field after changing the value.
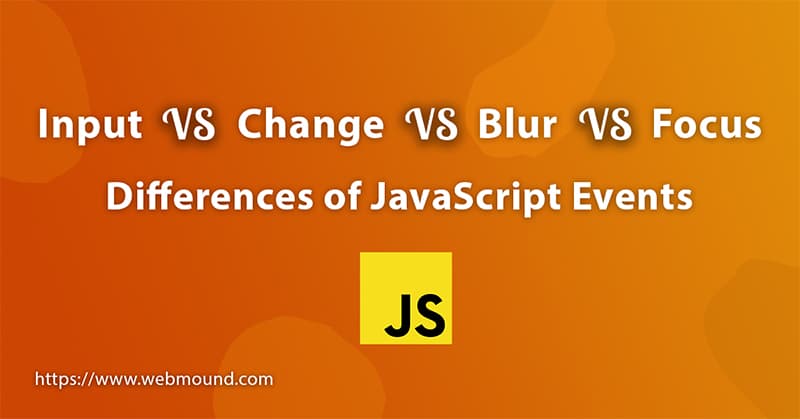
Blur VS Change Event in JavaScript
JavaScript blur
event fires whenever someone loses focus from an HTML input
field. It happens every time even if the value in that input
field does not change.
const username = document.getElementById('username');
username.addEventListener('blur', () => {
// Write your logic here
console.log('blur event');
});
On the other hand, the change
event fires only if the value in the input
field changes.
This is the main difference between blur
and change
events in JavaScript. Other than that, both events work similarly in terms of functionality.
For example, if the input
field contains the value "john" as username, I don't change the username but remove the focus from the field. In this case, the only blur
event will fire but the change
event will do nothing.
But if I change the username to "john_doe", both events will fire and execute the function.
So, the change
event will be a good choice if your logic needs to run when the value changes.
const username = document.getElementById('username');
username.addEventListener('change', () => {
// Write your logic here
console.log('change event');
});
Focus VS Blur: When to Use Focus and Blur Events in JavaScript
In JavaScript, the focus
and blur
events are opposite to each other. The focus
event occurs when you click on an input
field and the field gets focused.
The blur
event occurs when you click away from an input
field and the field loses focus.
- You can use the
focus
event if you need to run some logic whenever someone clicks on the input field. - You will use the blur event if you need to run some logic when the user leaves the input field by clicking away.
Conclusion
There are many JavaScript events we can use in our projects. Many of them are interchangeable with one another.
But as a developer, we must know the differences between these events. Then we will be able to use them properly in our applications.
In this article, I have discussed the following:
- Difference between input and change events in JavaScript.
- Difference between blur and change events in JavaScript.
- When to use focus and blur events and their difference in JavaScript.