When we have SSL or TLS certificates on our website, we redirect HTTP requests to HTTPS so that our users can use the secured version. Having an HTTPS version of our web page is also a ranking factor in Google.
Express middleware function runs for every request and can check the protocol. If the incoming request is using the HTTP protocol, the middleware will redirect it to the HTTPS version using the res.redirect()
method.
We can also extract the URL from the request object in the Express framework. In this way, we will redirect our user to the same URL but with the HTTPS protocol.
Let's see how we redirect from HTTP to HTTPS in Express and Node.js with examples.
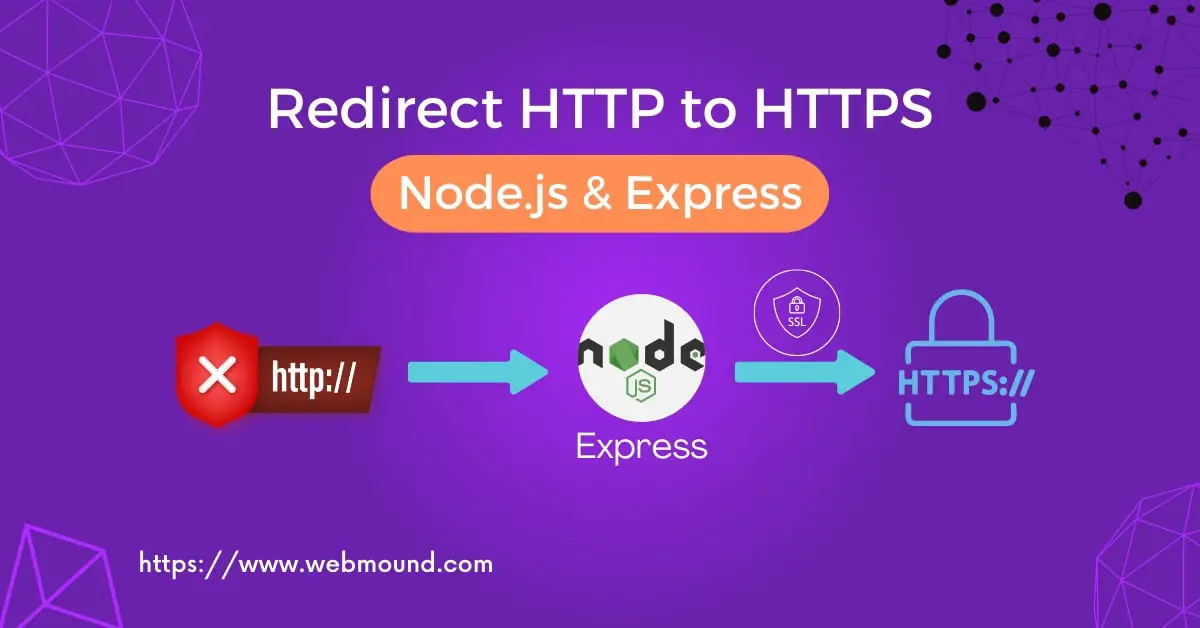
How to Redirect From HTTP to HTTPS in Express
I will use the Express middleware function because I want to redirect every HTTP request to HTTPS automatically. In this way, we can check the protocol for every request.
You have to add this middleware function before any other middleware functions and routes. It will make sure that we are checking the protocol before anything else.
app.use((req, res, next) => {
if (req.protocol === 'http') {
return res.redirect(301, `https://${req.headers.host}${req.url}`);
}
next();
});
I am checking if the protocol is equal to "http" using the req.protocol
property inside the middleware function. This property returns the protocol of the request.
If the incoming request is using an SSL certificate then this property will return "https" otherwise it will give "http".
When the condition is true, we will redirect to the HTTPS version. But before redirecting, we have to get the original URL from the req
object.
To construct the URL, you have to identify the host and URL path using req.headers.host
and req.url
properties respectively. Then I will redirect the URL in Express using res.redirect()
method.
There is another way to verify whether the request is using HTTPS or not. Instead of using the req.protocol
property, we can use the req.secure
property.
app.use((req, res, next) => {
if (!req.secure) {
return res.redirect(301, `https://${req.headers.host}${req.url}`);
}
next();
});
If the server has a TLS connection, this property returns true
otherwise false
. Therefore, if its value is false
that means the request is using HTTP.
In that case, we will redirect the request from HTTP to HTTPS with a 301 status code. You can use any of the 2 methods to identify the current protocol.
When a request comes with the HTTPS, the if
condition will not run and Express will handle the request as usual.
If we look at the complete code, it will look something like this:
const express = require('express');
const app = express();
app.use((req, res, next) => {
if (req.protocol === 'http') {
return res.redirect(301, `https://${req.headers.host}${req.url}`);
}
next();
});
app.get('/', (req, res) => {
res.send('<h1>Home Page</h1>');
});
app.get('/about', (req, res) => {
res.send('<h1>About Page</h1>');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Also Read: Easiest Way to Set & Use Environment Variables (.env) in Node.js
Conclusion
It is very easy to redirect from your Node.js server. You can perform any type of redirect using the Express framework.
But if you consider the performance, it will be better to redirect HTTP to HTTPS using Nginx or Apache web server software. It will be very fast and efficient.
But if you don't have access to that server software, you can redirect from HTTP to HTTPS using your Node.js and Express server.