To know more about Node.js runtime, we should have knowledge about creating a web server using the core module of NodeJS without using the frameworks.
Node.js has a built-in HTTP module to spin up a web server from scratch. This module provides a createServer()
method that takes a callback function to execute and returns a Server object. The listen()
method of this object is used to listen to the server at a specific port.
There are many Node.js frameworks out there. These frameworks give us easy access to the server by doing all the heavy lifting in the background. That's why these frameworks are used in almost every project to save time and energy.
But as Node.js developers, we should have core knowledge about this runtime and how it works. That's why in this article, we will see how to create a web server using the Node.js HTTP module.
Creating a Web Server in Node.js HTTP Module
There are 2 modules in Node.js that we can use to spin up our server. These are HTTP and HTTPS. They both create servers in Node.js but there is a difference between them.
The HTTPS module requires an SSL certificate to run a web server. Because it maintains encrypted communication between a user and the server. Without an SSL certificate, you can't use this module.
In this post, we will develop our server using the HTTP module. But you can also use the HTTPS module to create a Node.js server for production.
It doesn't mean using the HTTP module is a bad choice. You can add your SSL certificate when you deploy the server to a hosting provider. Nowadays, most hosting providers offer free SSL certificates.
We will create our Node.js server in just 3 simple steps. These are:
- Step 1: Create a Project Folder and JavaScript File.
- Step 2: Write The Node.js Server Instance.
- Step 3: Run The Node.js Server.
Before starting to develop the server, you need to have Node.js installed on your computer. I assume you have it in your system already.
Let's look into those steps in detail with examples.
Also Read: Best Setup to Use TypeScript with Node.js and Express Project
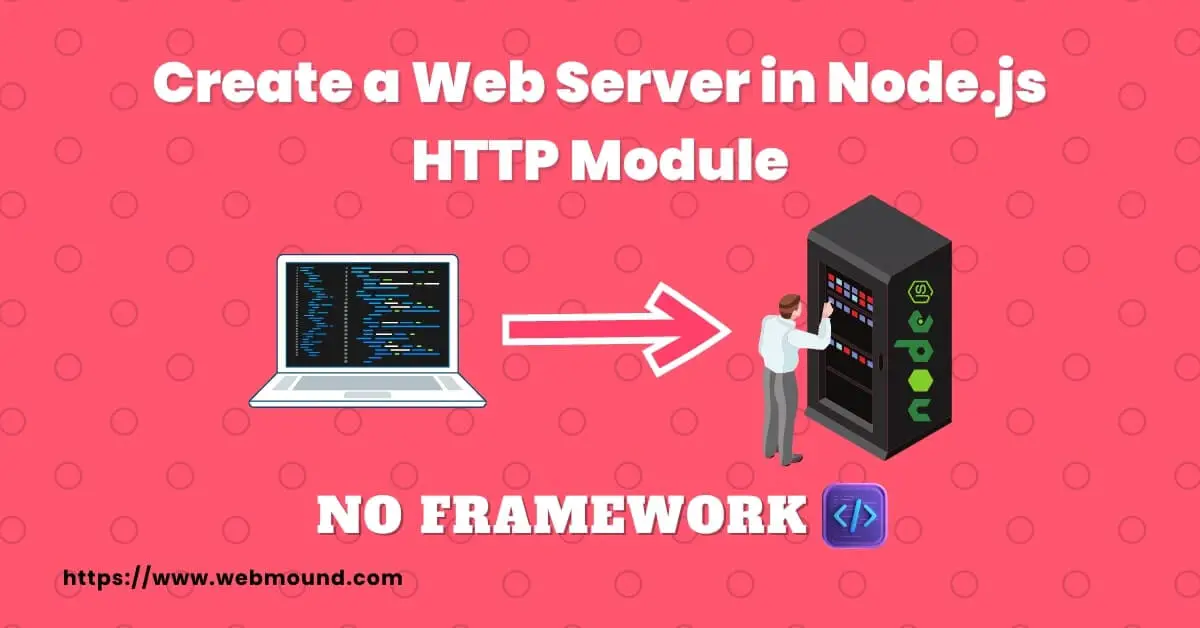
Step 1: Create a Project Folder and JavaScript File
At first, we need a folder where our server files will live. Then we will create a JavaScript file inside that folder. This will be the entry file for our server.
It will contain the code responsible to spin up our Node.js server. Run the following command in your terminal/command line to create a folder.
mkdir node-server
This command will create a folder called "node-server" inside your computer. You can name this folder whatever you want.
Go inside this folder using the following command.
cd node-server
After going inside the folder, we will create the JavaScript file for our server. You can use any name for this file. But it is common to use app.js or server.js in the Node.js community.
touch server.js
I am using server.js for the file name. Now open this "node-server" folder in your favorite code editor. Congratulations, you have completed step 1 successfully.
Also Read: Easy Ways to Redirect URL in Node.js (Express & HTTP Module)
Step 2: Write The Node.js Server Instance
In this step, we will do the most important part. We will write our Node.js server instance. You have to import the http
module from Node.js in the server.js file.
As it is a core module of Node.js runtime, therefore you don't need to install anything.
const http = require('http');
const server = http.createServer((req, res) => {
res.write('Welcome to the server');
res.end();
});
const port = 5000;
const hostname = 'localhost';
server.listen(port, hostname, () => {
console.log(`Server is running at http://${hostname}:${port}`);
});
After importing this module, you can execute the createServer()
method. This method takes a callback function.
Node.js automatically provides 2 parameters in this callback function. The first parameter is the request object and the second parameter is the response object.
req
- The request is a readable stream. This object contains all the necessary information about the request. You can extract the request type, URL, headers, etc. It offers many properties and methods to grab data from a request.res
- The response is a writable stream. That means we can write the necessary information on this stream and send it back to the user as a response. We usually set the HTTP status code, headers, contents, etc for the response.
I am writing plain text to the res
object by calling the write()
method. To send the response back to the user, you need to call the end()
method from the res
object.
The createServer()
method returns a Server
instance. By default, this server doesn't give a URL to access it. We have to listen to a specific port number using the listen()
method from the server instance.
The listen()
method can take up to 3 parameters. The first parameter defines the port number. Then the hostname and callback function are optional.
If you don't pass the hostname, it will be the "localhost" by default. Inside the callback function, you can display the success message or do anything else after listening to the server.
Also Read: Easiest Way to Set & Use Environment Variables (.env) in Node.js
Step 3: Run The Node.js Server
After writing the required code, it's time to execute the JavaScript file to spin up our Node.js server.
Open your terminal inside your server folder. I will open my terminal in my "node-server" folder. Because inside this folder, I have the server.js file.
node server.js
Output:
Server is running at http://localhost:5000
In your terminal, you will have access to the node
command. Because you have installed the Node.js runtime. Using this command, I can execute the server.js file to start my server.
Now, if I visit http://localhost:5000
in the browser, I will see the response on the screen.
What Else Can You Do in The Server?
Just sending a plain text as a response is not enough. We can attach many things with our response and extract from the request object to do something like routing.
You can also get the query string from an URL in the Node.js server.
Setting Status Code and Headers to the Response
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.write('Welcome to the server');
res.end();
});
const port = 5000;
const hostname = 'localhost';
server.listen(port, hostname, () => {
console.log(`Server is running at http://${hostname}:${port}`);
});
You can set the status code using statusCode
property from the res
object. I am setting 200 as the status code for the response. You can also use other status codes.
To set headers, the res object has the setHeader()
method. By calling this method, you can set any HTTP headers. I am sending plain text for this response, therefore I am setting the "Content-Type" header with the value "text/plain".
You can set multiple headers by calling the setHeader()
method multiple times.
How to Set Up Routes in Node.js Server
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/') {
// Home page
res.statusCode = 200;
res.setHeader('Content-Type', 'text/html');
res.write('<html><body><h1>Home Page</h1></body></html>');
return res.end();
} else if (req.url === '/about') {
// About page
res.statusCode = 200;
res.setHeader('Content-Type', 'text/html');
res.write('<html><body><h1>About Page</h1></body></html>');
return res.end();
}
// 404 Page
res.statusCode = 404;
res.setHeader('Content-Type', 'text/html');
res.write('<html><body><h1>Page not found</h1></body></html>');
res.end();
});
const port = 1000;
const hostname = 'localhost';
server.listen(port, hostname, () => {
console.log(`Server is running at http://${hostname}:${port}`);
});
To set up routes, you have to know which URL a user is visiting. You can get the URL data from the req.url
property.
Based on the URL, you can send a different response. In this example, I am setting routes for home and about pages. The server will return an HTML response for those routes.
If the server doesn't find any matching URL, it will return a 404-page response. As you can see, it is very easy to set up routes in a Node.js server without a framework.
It is important to notice that I am returning from every if
and else if
blocks. Because if the server finds a matching URL and sends the response, I don't want to run any other code.
If you try to run code after calling res.end()
method, the server will throw an error. Therefore, you have to return from every route.
Also Read: Best Guide to Download File Using Node.js (HTTPS Module)
How to Handle an Error in Server Connection
If you encounter any error while listening or running your Node.js server, you can handle that error using the "error" event.
If the Node.js server finds an error while starting the process, it emits an "error" event. We can catch that event by calling the on() method from the server object.
server.on('error', (err) => {
console.log(err);
});
The on()
method accepts the name of the event and a callback function in the second parameter. Inside the callback function, you have access to the Error object.
Now, you can handle this error however you want. You can access the message and name of the error using message
and name
property from th err
object.
Conclusion
We have developed a Node.js server that can handle user requests and send responses with appropriate status codes and headers.
You have seen how to set up routes and handle requests for multiple pages from your server. All of them are possible just by using Node.js core modules.
In this way, we can understand how the Node.js server really works under the hood. Even if we use a framework for our projects, this understanding will help us to use all the features of a framework properly.
I hope it was helpful for you. You have understood how to create a web server in Node.js HTTP module without using a framework from this tutorial.